So far we only have STATIC images with these lines. Let's use the USER's mouse position to stand in for one of the points:
line(mouseX,mouseY,250,150);
Make sure to pay attention to case sensitivity. As you will see, the command mouseX and mouseY will use the X and Y mouse position. If we insert the above line of code into our program then we only get that line once. What if we want to constantly get new lines...to have Processing actively update?
This is where we introduce a new concept...functions. Next we will use two simple functions that work very differently.
A FUNCTION is a set of lines of code that can be can be called over and over again. Here we will use two already defined functions. One is called setup() and the other is called draw(). It is easy to remember that setup() only runs once. This is where you can initialize settings such as size(x,y) or stroke(x).
The function draw() on the other hand runs repeatedly at frame rate. The default framerate is 60 frames per second.
We will start writing our function with the word void because our functions will not return any values. Also, after the open and close parentheses that follow the function name, we will open a curly bracket like this: {
After the curly bracket you can put as many lines of code as you wish. When you are finished writing your lines of code, you can CLOSE THE FUNCTION with a close curly bracket symbols like this: }
If this sounds confusing, look at the syntax (or format) of the following code:
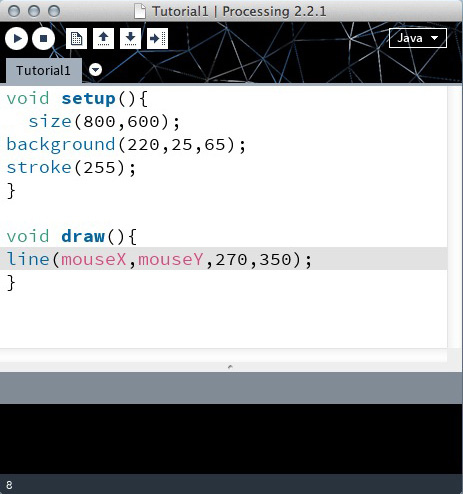
Now that you know this, see how we can create an interactive sketch? One end of each line draws where you mouse is and the other end draws at 270 pixels in the X-axis and 350 pixels down in the Y-axis. This operation repeats at 60 frames per second. Example result:
Once you try this out, try adding more lines of code to make the sketch more exciting.
Here is ONE example of how to make the image look better.
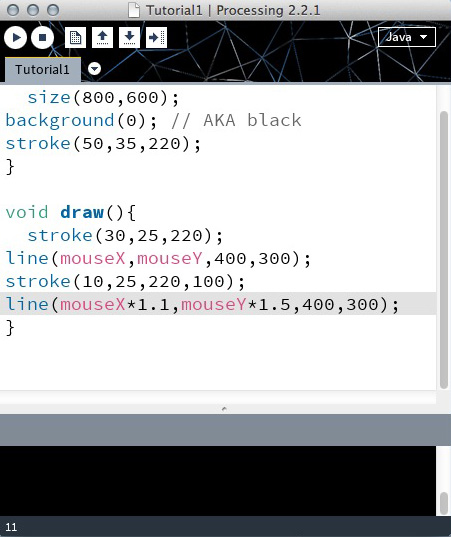
The above code makes some changes. One of the them is the stroke. If you put 3 different number values followed by commas, Processing knows you are talking about an RGB value. If you put a fourth value in their it knows you are talking about an alpha channel value (or transparency). So by using a stroke value of stroke(10, 25, 220, 100); I am saying a line that is 10 Red, 25 Green, 220 Blue and 100 Alpha. All of these values range from 0-255 (8 bit numbers).
I also changed the background() value to 0 to make a black backdrop. This should make foreground colors pop.
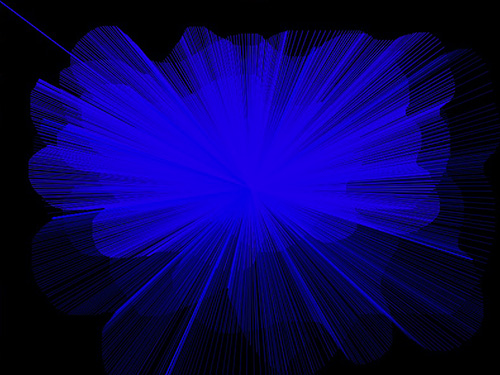
Try your own variations. Remember that there is no harm in experimenting...in fact it's the best way to learn. |