Now we will look at a little Sketch that uses a float value to change the rotation of an ellipse with every frame. The code looks like this:
//**********************
float rval=0;
void setup(){
size(1200,800);
background(0);
stroke(0,0,225,45);
smooth();
noFill();
}
void draw(){
rval += .05;
translate(mouseX,mouseY);
rotate(rval);
ellipse(0,0,80,190);
line(150, 25, 270, 350);
}
//**********************
or in the Processing window: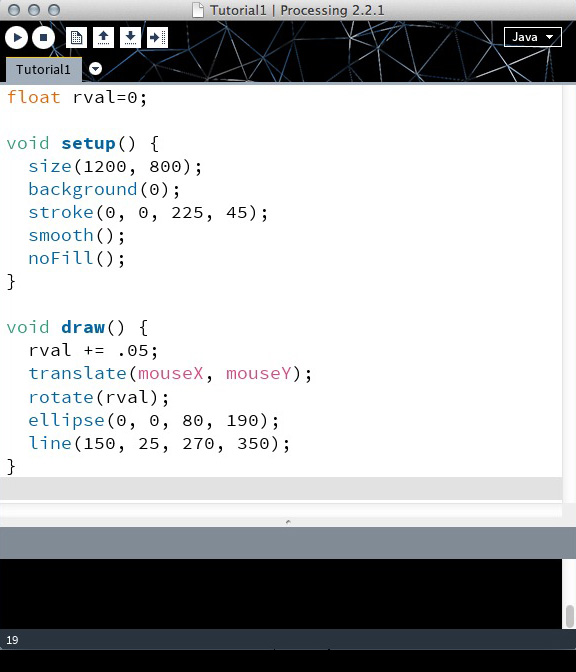
Try running the program to see how it looks and then start to see if you can figure out what it happening on your own. If you encounter a command that you don't recognize (the blue text lines means the word is a built-in command of the language). By RIGHT CLICKING on the selected text and by choosing 'Find In Reference' you can learn a surpising amount about this language.
New code to learn about:
translate();
translate is the same as "move" or "slide". By default processing is looking for two values...the first is the X-axis movement, or a LEFT/RIGHT slide. The second value is a Y-axis movement, or UP/DOWN slide.
The translate command moves the entire sketch canvas and all of its content that follow this command by the designated amount. All code above a translate() are NOT AFFECTED. All code after the translate() are affected. Values are in pixels.
rotate();
rotate(); rotates the entire sketch canvas
and all of its content that follows the rotate() call. There are two important catches. Processing always rotates content around the canvas origin, or 0,0 point. This is usually at the top left of sketch. The second important note is that processing rotates in terms of radians, not degrees. There are 2*PI radians in a circle (6.2831 radians in 360 degrees). If you want to automatically convert degrees to radians, use the radians() command. For example rotate(radians(45)); will convert 45 degrees to radians, then rotate by that amount.
By combining the translate() with the rotate() in the below sketch, we are moving the origin point (originally 0,0) to where the mouse is located. Then we are rotating around that point.
Below is an explanation via commented code.
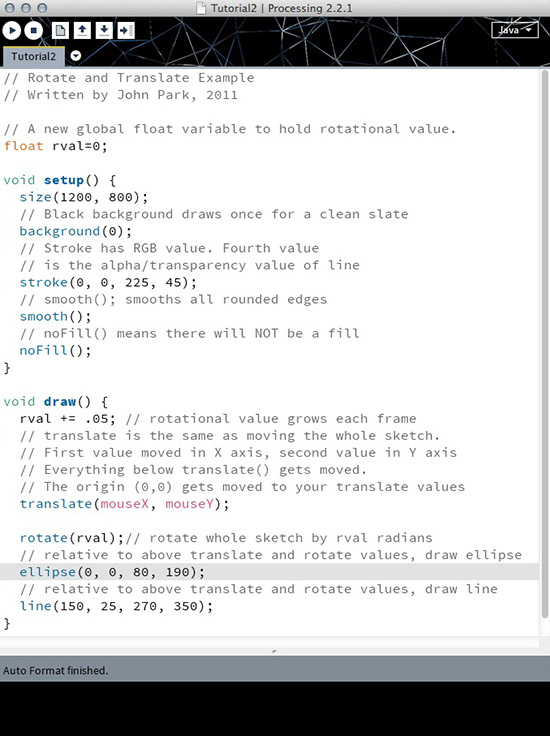
Try taking these skills and adding other shapes inside the draw function.
Other built-in shapes can be found in the REFENCE page for Processing under '2D Primitives' |